Blazorators by David Pine
Nuget / site data
Details
Info
Name: Blazorators
The source generator used to generate extension methods on the IJSInProcessRuntime type for WebAssembly JavaScript interop.
Author: David Pine
NuGet: https://www.nuget.org/packages/Blazor.SourceGenerators/
You can find more details at https://github.com/IEvangelist/blazorators/
Original Readme
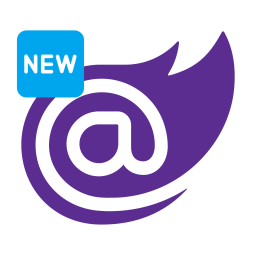
Thank you for perusing my Blazor C# Source Generator repository. I'd really appreciate a ⭐ if you find this interesting.
A C# source generator that creates fully functioning Blazor JavaScript interop code, targeting either the IJSInProcessRuntime
or IJSRuntime
types. This library provides several NuGet packages:
Core libraries
NuGet package | NuGet version | Description |
---|---|---|
Blazor.SourceGenerators | Core source generator library. | |
Blazor.Serialization | Common serialization library, required in some scenarios when using generics. |
WebAssembly libraries
NuGet package | NuGet version | Description |
---|---|---|
Blazor.LocalStorage.WebAssembly | Blazor WebAssembly class library exposing DI-ready IStorageService type for the localStorage implementation (relies on IJSInProcessRuntime ). | |
Blazor.SessionStorage.WebAssembly | Blazor WebAssembly class library exposing DI-ready IStorageService type for the sessionStorage implementation (relies on IJSInProcessRuntime ). | |
Blazor.Geolocation.WebAssembly | Razor class library exposing DI-ready IGeolocationService type (and dependent callback types) for the geolocation implementation (relies on IJSInProcessRuntime ). | |
Blazor.SpeechSynthesis.WebAssembly | Razor class library exposing DI-ready ISpeechSynthesisService type for the speechSynthesis implementation (relies on IJSInProcessRuntime ). |
Targets the
IJSInProcessRuntime
type.
Server libraries
NuGet package | NuGet version | Description |
---|---|---|
Blazor.LocalStorage | Blazor Server class library exposing DI-ready IStorageService type for the localStorage implementation (relies on IJSRuntime ) | |
Blazor.SessionStorage | Blazor Server class library exposing DI-ready IStorageService type for the sessionStorage implementation (relies on IJSRuntime ) | |
Blazor.Geolocation | Razor class library exposing DI-ready IGeolocationService type (and dependent callback types) for the geolocation implementation (relies on IJSRuntime ). | |
Blazor.SpeechSynthesis | Razor class library exposing DI-ready ISpeechSynthesisService type for the speechSynthesis implementation (relies on IJSRuntime ). |
Targets the
IJSRuntime
type.
Note
The reason that I generate two separate packages, one with an async API and another with the synchronous version is due to the explicit usage ofIJSInProcessRuntime
when using Blazor WebAssembly. This decision allows the APIs to be separate, and easily consumable from their repsective consuming Blazor apps, either Blazor server or Blazor WebAssembly. I might change it later to make this a consumer configuration, in that each consuming library will have to explicitly define a preprocessor directive to specifyIS_WEB_ASSEMBLY
defined.
Using the Blazor.SourceGenerators
package 📦
As an example, the official Blazor.LocalStorage.WebAssembly
package consumes the Blazor.SourceGenerators
package. It exposes extension methods specific to Blazor WebAssembly and the localStorage
Web API.
Consider the IStorageService.cs C# file:
// Copyright (c) David Pine. All rights reserved.
// Licensed under the MIT License.
namespace Microsoft.JSInterop;
[JSAutoGenericInterop(
TypeName = "Storage",
Implementation = "window.localStorage",
Url = "https://developer.mozilla.org/docs/Web/API/Window/localStorage",
GenericMethodDescriptors = new[]
{
"getItem",
"setItem:value"
})]
public partial interface IStorageService
{
}
This code designates itself into the Microsoft.JSInterop
namespace, making the source generated implementation available to anyone consumer who uses types from this namespace. It uses the JSAutoGenericInterop
to specify:
TypeName = "Storage"
: sets the type toStorage
.Implementation = "window.localStorage"
: expresses how to locate the implementation of the specified type from the globally scopedwindow
object, this is thelocalStorage
implementation.Url
: sets the URL for the implementation.GenericMethodDescriptors
: Defines the methods that should support generics as part of their source-generation. ThelocalStorage.getItem
is specified to return a genericTResult
type, and thelocalStorage.setItem
has its parameter with a name ofvalue
specified as a genericTArg
type.
The generic method descriptors syntax is:
"methodName"
for generic return type and"methodName:parameterName"
for generic parameter type.
The file needs to define an interface and it needs to be partial
, for example; public partial interface
. Decorating the class with the JSAutoInterop
(or `JSAutoGenericInterop) attribute will source generate the following C# code, as shown in the source generated IStorageServiceService.g.cs:
using Blazor.Serialization.Extensions;
using System.Text.Json;
#nullable enable
namespace Microsoft.JSInterop;
/// <summary>
/// Source generated interface definition of the <c>Storage</c> type.
/// </summary>
public partial interface IStorageServiceService
{
/// <summary>
/// Source generated implementation of <c>window.localStorage.length</c>.
/// <a href="https://developer.mozilla.org/docs/Web/API/Storage/length"></a>
/// </summary>
double Length
{
get;
}
/// <summary>
/// Source generated implementation of <c>window.localStorage.clear</c>.
/// <a href="https://developer.mozilla.org/docs/Web/API/Storage/clear"></a>
/// </summary>
void Clear();
/// <summary>
/// Source generated implementation of <c>window.localStorage.getItem</c>.
/// <a href="https://developer.mozilla.org/docs/Web/API/Storage/getItem"></a>
/// </summary>
TValue? GetItem<TValue>(string key, JsonSerializerOptions? options = null);
/// <summary>
/// Source generated implementation of <c>window.localStorage.key</c>.
/// <a href="https://developer.mozilla.org/docs/Web/API/Storage/key"></a>
/// </summary>
string? Key(double index);
/// <summary>
/// Source generated implementation of <c>window.localStorage.removeItem</c>.
/// <a href="https://developer.mozilla.org/docs/Web/API/Storage/removeItem"></a>
/// </summary>
void RemoveItem(string key);
/// <summary>
/// Source generated implementation of <c>window.localStorage.setItem</c>.
/// <a href="https://developer.mozilla.org/docs/Web/API/Storage/setItem"></a>
/// </summary>
void SetItem<TValue>(string key, TValue value, JsonSerializerOptions? options = null);
}
These internal extension methods rely on the IJSInProcessRuntime
to perform JavaScript interop. From the given TypeName
and corresponding Implementation
, the following code is also generated:
IStorageService.g.cs
: The interface for the correspondingStorage
Web API surface area.LocalStorgeService.g.cs
: Theinternal
implementation of theIStorageService
interface.LocalStorageServiceCollectionExtensions.g.cs
: Extension methods to add theIStorageService
service to the dependency injectionIServiceCollection
.
Here is the source generated LocalStorageService
implementation:
// Copyright (c) David Pine. All rights reserved.
// Licensed under the MIT License:
// https://github.com/IEvangelist/blazorators/blob/main/LICENSE
// Auto-generated by blazorators.
#nullable enable
using Blazor.Serialization.Extensions;
using Microsoft.JSInterop;
using System.Text.Json;
namespace Microsoft.JSInterop;
/// <inheritdoc />
internal sealed class LocalStorageService : IStorageService
{
private readonly IJSInProcessRuntime _javaScript = null;
/// <inheritdoc cref="P:Microsoft.JSInterop.IStorageService.Length" />
double IStorageService.Length => _javaScript.Invoke<double>("eval", new object[1]
{
"window.localStorage.length"
});
public LocalStorageService(IJSInProcessRuntime javaScript)
{
_javaScript = javaScript;
}
/// <inheritdoc cref="M:Microsoft.JSInterop.IStorageService.Clear" />
void IStorageService.Clear()
{
_javaScript.InvokeVoid("window.localStorage.clear");
}
/// <inheritdoc cref="M:Microsoft.JSInterop.IStorageService.GetItem``1(System.String,System.Text.Json.JsonSerializerOptions)" />
TValue? IStorageService.GetItem<TValue>(string key, JsonSerializerOptions? options)
{
return _javaScript.Invoke<string>("window.localStorage.getItem", new object[1]
{
key
}).FromJson<TValue>(options);
}
/// <inheritdoc cref="M:Microsoft.JSInterop.IStorageService.Key(System.Double)" />
string? IStorageService.Key(double index)
{
return _javaScript.Invoke<string>("window.localStorage.key", new object[1]
{
index
});
}
/// <inheritdoc cref="M:Microsoft.JSInterop.IStorageService.RemoveItem(System.String)" />
void IStorageService.RemoveItem(string key)
{
_javaScript.InvokeVoid("window.localStorage.removeItem", key);
}
/// <inheritdoc cref="M:Microsoft.JSInterop.IStorageService.SetItem``1(System.String,``0,System.Text.Json.JsonSerializerOptions)" />
void IStorageService.SetItem<TValue>(string key, TValue value, JsonSerializerOptions? options)
{
_javaScript.InvokeVoid("window.localStorage.setItem", key, value.ToJson<TValue>(options));
}
}
Finally, here is the source generated service collection extension methods:
using Microsoft.JSInterop;
namespace Microsoft.Extensions.DependencyInjection;
/// <summary></summary>
public static class LocalStorageServiceCollectionExtensions
{
/// <summary>
/// Adds the <see cref="IStorageService" /> service to the service collection.
/// </summary>
public static IServiceCollection AddLocalStorageServices(
this IServiceCollection services) =>
services.AddSingleton<IJSInProcessRuntime>(serviceProvider =>
(IJSInProcessRuntime)serviceProvider.GetRequiredService<IJSRuntime>())
.AddSingleton<IStorageService, LocalStorageService>();
}
Putting this all together, the Blazor.LocalStorage.WebAssembly
NuGet package is actually less than 15 lines of code, and it generates full DI-ready services with JavaScript interop.
The Blazor.LocalStorage
package, generates extensions on the IJSRuntime
type.
// Copyright (c) David Pine. All rights reserved.
// Licensed under the MIT License.
namespace Microsoft.JSInterop;
[JSAutoInterop(
TypeName = "Storage",
Implementation = "window.localStorage",
HostingModel = BlazorHostingModel.Server,
OnlyGeneratePureJS = true,
Url = "https://developer.mozilla.org/docs/Web/API/Window/localStorage")]
public partial interface IStorageServiceService
{
}
Generates the following:
// Copyright (c) David Pine. All rights reserved.
// Licensed under the MIT License:
// https://github.com/IEvangelist/blazorators/blob/main/LICENSE
// Auto-generated by blazorators.
using System.Threading.Tasks;
#nullable enable
namespace Microsoft.JSInterop;
public partial interface IStorageServiceService
{
/// <summary>
/// Source generated implementation of <c>window.localStorage.length</c>.
/// <a href="https://developer.mozilla.org/docs/Web/API/Storage/length"></a>
/// </summary>
ValueTask<double> Length
{
get;
}
/// <summary>
/// Source generated implementation of <c>window.localStorage.clear</c>.
/// <a href="https://developer.mozilla.org/docs/Web/API/Storage/clear"></a>
/// </summary>
ValueTask ClearAsync();
/// <summary>
/// Source generated implementation of <c>window.localStorage.getItem</c>.
/// <a href="https://developer.mozilla.org/docs/Web/API/Storage/getItem"></a>
/// </summary>
ValueTask<string?> GetItemAsync(string key);
/// <summary>
/// Source generated implementation of <c>window.localStorage.key</c>.
/// <a href="https://developer.mozilla.org/docs/Web/API/Storage/key"></a>
/// </summary>
ValueTask<string?> KeyAsync(double index);
/// <summary>
/// Source generated implementation of <c>window.localStorage.removeItem</c>.
/// <a href="https://developer.mozilla.org/docs/Web/API/Storage/removeItem"></a>
/// </summary>
ValueTask RemoveItemAsync(string key);
/// <summary>
/// Source generated implementation of <c>window.localStorage.setItem</c>.
/// <a href="https://developer.mozilla.org/docs/Web/API/Storage/setItem"></a>
/// </summary>
ValueTask SetItemAsync(string key, string value);
}
Notice, that since the generic method descriptors are not added generics are not supported. This is not yet implemented as I've been focusing on WebAssembly scenarios.
Design goals 🎯
I was hoping to use the TypeScript lib.dom.d.ts bits as input. This input would be read, parsed, and cached within the generator. The generator code would be capable of generating extension methods on the IJSRuntime
. Additionally, the generator will create object graphs from the well know web APIs.
Using the lib.dom.d.ts file, we could hypothetically parse various TypeScript type definitions. These definitions could then be converted to C# counterparts. While I realize that not all TypeScript is mappable to C#, there is a bit of room for interpretation.
Consider the following type definition:
/**
An object can programmatically obtain the position of the device.
It gives Web content access to the location of the device. This allows
a Web site or app to offer customized results based on the user's location.
*/
interface Geolocation {
clearWatch(watchId: number): void;
getCurrentPosition(
successCallback: PositionCallback,
errorCallback?: PositionErrorCallback | null,
options?: PositionOptions): void;
watchPosition(
successCallback: PositionCallback,
errorCallback?: PositionErrorCallback | null,
options?: PositionOptions): number;
}
This is from the TypeScript repo, lib.dom.d.ts file lines 5,498-5,502.
Example consumption of source generator ✔️
Ideally, I would like to be able to define a C# class such as this:
// Copyright (c) David Pine. All rights reserved.
// Licensed under the MIT License.
namespace Microsoft.JSInterop;
[JSAutoInterop(
TypeName = "Geolocation",
Implementation = "window.navigator.geolocation",
Url = "https://developer.mozilla.org/docs/Web/API/Geolocation")]
public partial interface IGeolocationService
{
}
The source generator will expose the JSAutoInteropAttribute
, and consuming libraries will decorate their classes with it. The generator code will see this class, and use the TypeName
from the attribute to find the corresponding type to implement.
With the type name, the generator will generate the corresponding methods, and return types. The method implementations will be extensions of the IJSRuntime
.
The following is an example resulting source generated IGeolocationService
object:
namespace Microsoft.JSInterop;
public partial interface IGeolocationService
{
/// <summary>
/// Source generated implementation of <c>window.navigator.geolocation.clearWatch</c>.
/// <a href="https://developer.mozilla.org/docs/Web/API/Geolocation/clearWatch"></a>
/// </summary>
void ClearWatch(double watchId);
/// <summary>
/// Source generated implementation of <c>window.navigator.geolocation.getCurrentPosition</c>.
/// <a href="https://developer.mozilla.org/docs/Web/API/Geolocation/getCurrentPosition"></a>
/// </summary>
/// <param name="component">The calling Razor (or Blazor) component.</param>
/// <param name="onSuccessCallbackMethodName">Expects the name of a <c>"JSInvokableAttribute"</c> C# method with the following <c>System.Action{GeolocationPosition}"</c>.</param>
/// <param name="onErrorCallbackMethodName">Expects the name of a <c>"JSInvokableAttribute"</c> C# method with the following <c>System.Action{GeolocationPositionError}"</c>.</param>
/// <param name="options">The <c>PositionOptions</c> value.</param>
void GetCurrentPosition<TComponent>(
TComponent component,
string onSuccessCallbackMethodName,
string? onErrorCallbackMethodName = null,
PositionOptions? options = null)
where TComponent : class;
/// <summary>
/// Source generated implementation of <c>window.navigator.geolocation.watchPosition</c>.
/// <a href="https://developer.mozilla.org/docs/Web/API/Geolocation/watchPosition"></a>
/// </summary>
/// <param name="component">The calling Razor (or Blazor) component.</param>
/// <param name="onSuccessCallbackMethodName">Expects the name of a <c>"JSInvokableAttribute"</c> C# method with the following <c>System.Action{GeolocationPosition}"</c>.</param>
/// <param name="onErrorCallbackMethodName">Expects the name of a <c>"JSInvokableAttribute"</c> C# method with the following <c>System.Action{GeolocationPositionError}"</c>.</param>
/// <param name="options">The <c>PositionOptions</c> value.</param>
double WatchPosition<TComponent>(
TComponent component,
string onSuccessCallbackMethodName,
string? onErrorCallbackMethodName = null,
PositionOptions? options = null)
where TComponent : class;
}
The generator will also produce the corresponding APIs object types. For example, the Geolocation API defines the following:
GeolocationService
PositionOptions
GeolocationCoordinates
GeolocationPosition
GeolocationPositionError
namespace Microsoft.JSInterop;
/// <inheritdoc />
internal sealed class GeolocationService : IGeolocationService
{
private readonly IJSInProcessRuntime _javaScript = null;
public GeolocationService(IJSInProcessRuntime javaScript)
{
_javaScript = javaScript;
}
/// <inheritdoc cref="M:Microsoft.JSInterop.IGeolocationService.ClearWatch(System.Double)" />
void IGeolocationService.ClearWatch(double watchId)
{
_javaScript.InvokeVoid("window.navigator.geolocation.clearWatch", watchId);
}
/// <inheritdoc cref="M:Microsoft.JSInterop.IGeolocationService.GetCurrentPosition``1(``0,System.String,System.String,Microsoft.JSInterop.PositionOptions)" />
void IGeolocationService.GetCurrentPosition<TComponent>(
TComponent component,
string onSuccessCallbackMethodName,
string? onErrorCallbackMethodName,
PositionOptions? options)
{
_javaScript.InvokeVoid("blazorators.getCurrentPosition", DotNetObjectReference.Create<TComponent>(component), onSuccessCallbackMethodName, onErrorCallbackMethodName, options);
}
/// <inheritdoc cref="M:Microsoft.JSInterop.IGeolocationService.WatchPosition``1(``0,System.String,System.String,Microsoft.JSInterop.PositionOptions)" />
double IGeolocationService.WatchPosition<TComponent>(
TComponent component,
string onSuccessCallbackMethodName,
string? onErrorCallbackMethodName,
PositionOptions? options)
{
return _javaScript.Invoke<double>("blazorators.watchPosition", new object[4]
{
DotNetObjectReference.Create<TComponent>(component),
onSuccessCallbackMethodName,
onErrorCallbackMethodName,
options
});
}
}
using System.Text.Json.Serialization;
namespace Microsoft.JSInterop;
/// <summary>
/// Source-generated object representing an ideally immutable <c>GeolocationPosition</c> value.
/// </summary>
public class GeolocationPosition
{
/// <summary>
/// Source-generated property representing the <c>GeolocationPosition.coords</c> value.
/// </summary>
[JsonPropertyName("coords")]
public GeolocationCoordinates Coords
{
get;
set;
}
/// <summary>
/// Source-generated property representing the <c>GeolocationPosition.timestamp</c> value.
/// </summary>
[JsonPropertyName("timestamp")]
public long Timestamp
{
get;
set;
}
/// <summary>
/// Source-generated property representing the <c>GeolocationPosition.timestamp</c> value,
/// converted as a <see cref="T:System.DateTime" /> in UTC.
/// </summary>
[JsonIgnore]
public DateTime TimestampAsUtcDateTime => Timestamp.ToDateTimeFromUnix();
}
/// <summary>
/// Source-generated object representing an ideally immutable <c>GeolocationCoordinates</c> value.
/// </summary>
public class GeolocationCoordinates
{
/// <summary>
/// Source-generated property representing the <c>GeolocationCoordinates.accuracy</c> value.
/// </summary>
[JsonPropertyName("accuracy")]
public double Accuracy
{
get;
set;
}
/// <summary>
/// Source-generated property representing the <c>GeolocationCoordinates.altitude</c> value.
/// </summary>
[JsonPropertyName("altitude")]
public double? Altitude
{
get;
set;
}
/// <summary>
/// Source-generated property representing the <c>GeolocationCoordinates.altitudeAccuracy</c> value.
/// </summary>
[JsonPropertyName("altitudeAccuracy")]
public double? AltitudeAccuracy
{
get;
set;
}
/// <summary>
/// Source-generated property representing the <c>GeolocationCoordinates.heading</c> value.
/// </summary>
[JsonPropertyName("heading")]
public double? Heading
{
get;
set;
}
/// <summary>
/// Source-generated property representing the <c>GeolocationCoordinates.latitude</c> value.
/// </summary>
[JsonPropertyName("latitude")]
public double Latitude
{
get;
set;
}
/// <summary>
/// Source-generated property representing the <c>GeolocationCoordinates.longitude</c> value.
/// </summary>
[JsonPropertyName("longitude")]
public double Longitude
{
get;
set;
}
/// <summary>
/// Source-generated property representing the <c>GeolocationCoordinates.speed</c> value.
/// </summary>
[JsonPropertyName("speed")]
public double? Speed
{
get;
set;
}
}
/// <summary>
/// Source-generated object representing an ideally immutable <c>GeolocationPositionError</c> value.
/// </summary>
public class GeolocationPositionError
{
/// <summary>
/// Source-generated property representing the <c>GeolocationPositionError.code</c> value.
/// </summary>
[JsonPropertyName("code")]
public double Code
{
get;
set;
}
/// <summary>
/// Source-generated property representing the <c>GeolocationPositionError.message</c> value.
/// </summary>
[JsonPropertyName("message")]
public string Message
{
get;
set;
}
/// <summary>
/// Source-generated property representing the <c>GeolocationPositionError.PERMISSION_DENIED</c> value.
/// </summary>
[JsonPropertyName("PERMISSION_DENIED")]
public double PERMISSION_DENIED
{
get;
set;
}
/// <summary>
/// Source-generated property representing the <c>GeolocationPositionError.POSITION_UNAVAILABLE</c> value.
/// </summary>
[JsonPropertyName("POSITION_UNAVAILABLE")]
public double POSITION_UNAVAILABLE
{
get;
set;
}
/// <summary>
/// Source-generated property representing the <c>GeolocationPositionError.TIMEOUT</c> value.
/// </summary>
[JsonPropertyName("TIMEOUT")]
public double TIMEOUT
{
get;
set;
}
}
// Additional models omitted for brevity...
In addition to this GeolocationExtensions
class being generated, the generator will also generate a bit of JavaScript. Some methods cannot be directly invoked as they define callbacks. The approach the generator takes is to delegate callback methods on a given T
instance, with the JSInvokable
attribute. Our generator should also warn when the corresponding T
instance doesn't define a matching method name that is also JSInvokable
.
const getCurrentLocation =
(dotnetObj, successMethodName, errorMethodName, options) =>
{
if (navigator && navigator.geolocation) {
navigator.geolocation.getCurrentPosition(
(position) => {
dotnetObj.invokeMethodAsync(
successMethodName, position);
},
(error) => {
dotnetObj.invokeMethodAsync(
errorMethodName, error);
},
options);
}
};
// Other implementations omitted for brevity...
// But we'd also define a "watchPosition" wrapper.
// The "clearWatch" is a straight pass-thru, no wrapper needed.
window.blazorator = {
getCurrentLocation,
watchPosition
};
The resulting JavaScript will have to be exposed to consuming projects. Additionally, consuming projects will need to adhere to extension method consumption semantics. When calling generated extension methods that require .NET object references of type T
, the callback names should be marked with JSInvokable
and the nameof
operator should be used to ensure names are accurate. Consider the following example consuming Blazor component:
using Microsoft.AspNetCore.Components;
using Microsoft.JSInterop;
using Microsoft.JSInterop.Extensions;
namespace Example.Components;
// This is the other half of ConsumingComponent.razor
public sealed partial class ConsumingComponent
{
[Inject]
public IJSRuntime JavaScript { get; set; }
protected override async Task OnAfterRenderAsync(bool firstRender)
{
if (firstRender)
{
await JavaScript.GetCurrentPositionAsync(
this,
nameof(OnCoordinatesPermitted),
nameof(OnErrorRequestingCoordinates));
}
}
[JSInvokable]
public async Task OnCoordinatesPermitted(
GeolocationPosition position)
{
// TODO: consume/handle position.
await InvokeAsync(StateHasChanged);
}
[JSInvokable]
public async Task OnErrorRequestingCoordinates(
GeolocationPositionError error)
{
// TODO: consume/handle error.
await InvokeAsync(StateHasChanged);
}
}
Pseudocode and logical flow ➡️
- Consumer decorates a
static partial class
with theJSAutoInteropAttribute
. - Source generator is called:
JavaScriptInteropGenerator.Initialize
JavaScriptInteropGenerator.Execute
- The generator determines the
TypeName
from the attribute of the contextual class.- The
TypeName
is used to look up the corresponding TypeScript type definition. - If found, and a valid API - attempt source generation.
- The
Future work
- https://developer.mozilla.org/docs/Web/API/CredentialsContainer
- https://developer.mozilla.org/docs/Web/API/WakeLock
- https://developer.mozilla.org/docs/Web/API/Navigator/hid
- https://developer.mozilla.org/docs/Web/API/Web_Crypto_API
Known limitations ⚠️
At the time of writing, only pure JavaScript interop is supported. It is a stretch goal to add the following (currently missing) features:
- Source generate corresponding (and supporting) JavaScript files.
- We'd need to accept a desired output path from the consumer,
JavaScriptOutputPath
. - We would need to append all JavaScript into a single builder, and emit it collectively.
- We'd need to accept a desired output path from the consumer,
- Allow for declarative and custom type mappings, for example; suppose the consumer wants the API to use generics instead of
string
.- We'd need to expose a
TypeConverter
parameter and allow for consumers to implement their own. - We'd provide a default one for standard JSON serialization,
StringTypeConverter
(maybe make this the default).
- We'd need to expose a
References and resources 📑
- MDN Web Docs: Web APIs
- TypeScript DOM lib generator
- ASP.NET Core Docs: Blazor JavaScript interop
- Jared Parsons - GitHub Channel 9 Source Generators
- .NET Docs: C# Source Generators
- Source Generators Cookbook
- Source Generators: Design Document
Contributors ✨
Thanks goes to these wonderful people (emoji key):
Weihan Li 💻 | David Pine 💻 🎨 👀 🤔 ⚠️ | Robert McLaws 💻 🐛 🤔 | Colin Dembovsky 🚇 📦 | Tanay Parikh 📖 | Andreas Müller 🐛 💻 | Mahmudul Hasan 💻 |
fabiansanchez18 🐛 | Sean Feldman 🐛 | daver77 🤔 |
This project follows the all-contributors specification. Contributions of any kind are welcome!
About
Generate javascript interop code for Blazor WASM projects.
How to use
Example ( source csproj, source files )
- CSharp Project
- Program.cs
- ILocalStorageService.cs
- Home.razor
This is the CSharp Project that references Blazorators
<Project Sdk="Microsoft.NET.Sdk.BlazorWebAssembly">
<PropertyGroup>
<TargetFramework>net8.0</TargetFramework>
<Nullable>enable</Nullable>
<ImplicitUsings>enable</ImplicitUsings>
</PropertyGroup>
<ItemGroup>
<PackageReference Include="Microsoft.AspNetCore.Components.WebAssembly" Version="8.0.1" />
<PackageReference Include="Microsoft.AspNetCore.Components.WebAssembly.DevServer" Version="8.0.1" PrivateAssets="all" />
</ItemGroup>
<ItemGroup>
<PackageReference Include="Blazor.Serialization" Version="8.0.0" />
<PackageReference Include="Blazor.SourceGenerators"
Version="8.0.0"
OutputItemType="Analyzer"
SetTargetFramework="TargetFramework=netstandard2.0"
ReferenceOutputAssembly="false">
</PackageReference>
</ItemGroup>
<PropertyGroup>
<EmitCompilerGeneratedFiles>true</EmitCompilerGeneratedFiles>
<CompilerGeneratedFilesOutputPath>$(BaseIntermediateOutputPath)\GX</CompilerGeneratedFilesOutputPath>
</PropertyGroup>
</Project>
This is the use of Blazorators in Program.cs
using BlazorApp2;
using Microsoft.AspNetCore.Components.Web;
using Microsoft.AspNetCore.Components.WebAssembly.Hosting;
var builder = WebAssemblyHostBuilder.CreateDefault(args);
builder.RootComponents.Add<App>("#app");
builder.RootComponents.Add<HeadOutlet>("head::after");
builder.Services.AddScoped(sp => new HttpClient { BaseAddress = new Uri(builder.HostEnvironment.BaseAddress) });
builder.Services.AddLocalStorageServices();
await builder.Build().RunAsync();
This is the use of Blazorators in ILocalStorageService.cs
namespace BlazorApp2;
[JSAutoGenericInterop(
TypeName = "Storage",
Implementation = "window.localStorage",
Url = "https://developer.mozilla.org/docs/Web/API/Window/localStorage",
GenericMethodDescriptors = new[]
{
"getItem",
"setItem:value"
})]
public partial interface ILocalStorageService
{
}
This is the use of Blazorators in Home.razor
@page "/"
@inject ILocalStorageService MyLocalStorage;
<PageTitle>Home</PageTitle>
<h1>Hello, world!</h1>
Please refresh the page to see the value persisted in local storage.
@{
var name = MyLocalStorage.GetItem<string>("name");
if (name != null)
{
<p>Hi, @name</p>
}
else
{
<p>Hi, stranger</p>
}
MyLocalStorage.SetItem("name", "Andrei Ignat");
}
Generated Files
Those are taken from $(BaseIntermediateOutputPath)\GX
- BlazorHostingModel.g.cs
- ILocalStorageService.g.cs
- JSAutoGenericInteropAttribute.g.cs
- JSAutoInteropAttribute.g.cs
- LocalStorageService.g.cs
- LocalStorageServiceCollectionExtensions.g.cs
- RecordCompat.g.cs
/// <summary>
/// The Blazor hosting model source, either WebAssembly or Server.
/// </summary>
public enum BlazorHostingModel
{
/// <summary>
/// This is the default. Use this to source generate targeting the synchronous <c>IJSInProcessRuntime</c> type.
/// </summary>
WebAssembly,
/// <summary>
/// Use this to source generate targeting the synchronous <c>IJSRuntime</c> type.
/// </summary>
Server
};
// Copyright (c) David Pine. All rights reserved.
// Licensed under the MIT License:
// https://bit.ly/blazorators-license
// Auto-generated by blazorators.
using Blazor.Serialization.Extensions;
using System.Text.Json;
#nullable enable
namespace BlazorApp2;
/// <summary>
/// Source generated interface definition of the <c>Storage</c> type.
/// </summary>
public partial interface ILocalStorageService
{
/// <summary>
/// Source generated implementation of <c>window.localStorage.clear</c>.
/// <a href="https://developer.mozilla.org/docs/Web/API/Storage/clear"></a>
/// </summary>
void Clear();
/// <summary>
/// Source generated implementation of <c>window.localStorage.getItem</c>.
/// <a href="https://developer.mozilla.org/docs/Web/API/Storage/getItem"></a>
/// </summary>
TValue? GetItem<TValue>(string key, JsonSerializerOptions? options = null);
/// <summary>
/// Source generated implementation of <c>window.localStorage.key</c>.
/// <a href="https://developer.mozilla.org/docs/Web/API/Storage/key"></a>
/// </summary>
string? Key(double index);
/// <summary>
/// Source generated implementation of <c>window.localStorage.removeItem</c>.
/// <a href="https://developer.mozilla.org/docs/Web/API/Storage/removeItem"></a>
/// </summary>
void RemoveItem(string key);
/// <summary>
/// Source generated implementation of <c>window.localStorage.setItem</c>.
/// <a href="https://developer.mozilla.org/docs/Web/API/Storage/setItem"></a>
/// </summary>
void SetItem<TValue>(string key, TValue value, JsonSerializerOptions? options = null);
/// <summary>
/// Source generated implementation of <c>window.localStorage.length</c>.
/// <a href="https://developer.mozilla.org/docs/Web/API/Storage/length"></a>
/// </summary>
double Length { get; }
}
#nullable enable
/// <summary>
/// Use this attribute on <code>public static partial</code> extension method classes.
/// For example:
/// <example>
/// <code>
/// [JSAutoGenericInterop(
/// TypeName = "Storage",
/// Implementation = "window.localStorage",
/// HostingModel = BlazorHostingModel.WebAssembly,
/// Url = "https://developer.mozilla.org/docs/Web/API/Window/localStorage",
/// GenericMethodDescriptors = new[]
/// {
/// "getItem",
/// "setItem:value"
/// })]
/// public static partial LocalStorageExtensions
/// {
/// }
/// </code>
/// </example>
/// This will source generate all the extension methods for the IJSInProcessRuntime type for the localStorage APIs.
/// </summary>
public class JSAutoGenericInteropAttribute : JSAutoInteropAttribute
{
/// <summary>
/// The descriptors that define which APIs are to use default JSON serialization and support generics.
/// For example:
/// <code>
/// new[]
/// {
/// "getItem", // Serializes the return type of getItem as TValue
/// "setItem:value" // Serializes the value parameter of the setItem TValue
/// }
/// </code>
/// </summary>
public string[] GenericMethodDescriptors { get; set; } = null!;
/// <summary>
/// The overrides that define which APIs to override (only applicable for pure JavaScript).
/// For example:
/// <code>
/// new[]
/// {
/// "getVoices", // A pure JS method with by this name will have a custom impl.
/// }
/// </code>
/// </summary>
public string[] PureJavaScriptOverrides { get; set; } = null!;
}
#nullable enable
/// <summary>
/// Use this attribute on <code>public static partial</code> extension method classes.
/// For example:
/// <code>
/// [JSAutoInterop(
/// TypeName = "Storage",
/// Implementation = "window.localStorage",
/// HostingModel = BlazorHostingModel.WebAssembly,
/// Url = "https://developer.mozilla.org/docs/Web/API/Window/localStorage")]
/// public static partial LocalStorageExtensions
/// {
/// }
/// </code>
/// This will source generate all the extension methods for the IJSInProcessRuntime type for the localStorage APIs.
/// </summary>
[AttributeUsage(
AttributeTargets.Interface,
Inherited = false,
AllowMultiple = false)]
public class JSAutoInteropAttribute : Attribute
{
/// <summary>
/// The type name that corresponds to the lib.dom.d.ts interface. For example, <c>"Geolocation"</c>.
/// For more information, search 'interface {Name}'
/// <a href='https://raw.githubusercontent.com/microsoft/TypeScript/main/lib/lib.dom.d.ts'>here for types</a>.
/// </summary>
public string TypeName { get; set; } = null!;
/// <summary>
/// The path from the <c>window</c> object to the corresponding <see cref="TypeName"/> implementation.
/// For example, if the <see cref="TypeName"/> was <c>"Geolocation"</c>, this would be
/// <c>"window.navigator.geolocation"</c> (or <c>"navigator.geolocation"</c>).
/// </summary>
public string Implementation { get; set; } = null!;
/// <summary>
/// Whether to generate only pure JavaScript functions that do not require callbacks.
/// For example, <c>"Geolocation.clearWatch"</c> is consider pure, but <c>"Geolocation.watchPosition"</c> is not.
/// Defaults to <c>true</c>.
/// </summary>
public bool OnlyGeneratePureJS { get; set; } = true;
/// <summary>
/// The Blazor hosting model to generate source for. WebAssembly creates <c>IJSInProcessRuntime</c> extensions,
/// while Server creates <c>IJSRuntime</c> extensions. Defaults to <see cref="BlazorHostingModel.WebAssembly" />.
/// </summary>
public BlazorHostingModel HostingModel { get; set; } = BlazorHostingModel.WebAssembly;
/// <summary>
/// The optional URL to the corresponding API.
/// </summary>
public string? Url { get; set; }
/// <summary>
/// An optional array of TypeScript type declarations sources. Valid values are URLs or file paths.
/// </summary>
public string[]? TypeDeclarationSources { get; set; }
}
// Copyright (c) David Pine. All rights reserved.
// Licensed under the MIT License:
// https://bit.ly/blazorators-license
// Auto-generated by blazorators.
using Blazor.Serialization.Extensions;
using System.Text.Json;
#nullable enable
namespace BlazorApp2;
/// <inheritdoc/>
internal sealed class LocalStorageService : ILocalStorageService
{
internal readonly IJSInProcessRuntime _javaScript = null !;
public LocalStorageService(IJSInProcessRuntime javaScript) => _javaScript = javaScript;
/// <inheritdoc cref = "ILocalStorageService.Clear"/>
void ILocalStorageService.Clear() => _javaScript.InvokeVoid("window.localStorage.clear");
/// <inheritdoc cref = "ILocalStorageService.GetItem"/>
TValue? ILocalStorageService.GetItem<TValue>(string key, JsonSerializerOptions? options)
where TValue : default => _javaScript.Invoke<string?>("window.localStorage.getItem", key).FromJson<TValue>(options);
/// <inheritdoc cref = "ILocalStorageService.Key"/>
string? ILocalStorageService.Key(double index) => _javaScript.Invoke<string?>("window.localStorage.key", index);
/// <inheritdoc cref = "ILocalStorageService.RemoveItem"/>
void ILocalStorageService.RemoveItem(string key) => _javaScript.InvokeVoid("window.localStorage.removeItem", key);
/// <inheritdoc cref = "ILocalStorageService.SetItem"/>
void ILocalStorageService.SetItem<TValue>(string key, TValue value, JsonSerializerOptions? options) => _javaScript.InvokeVoid("window.localStorage.setItem", key, value.ToJson(options));
/// <inheritdoc cref = "ILocalStorageService.Length"/>
double ILocalStorageService.Length => _javaScript.Invoke<double>("eval", "window.localStorage.length");
}
// Copyright (c) David Pine. All rights reserved.
// Licensed under the MIT License:
// https://github.com/IEvangelist/blazorators/blob/main/LICENSE
// Auto-generated by blazorators.
using Microsoft.JSInterop;
namespace Microsoft.Extensions.DependencyInjection;
/// <summary></summary>
public static class LocalStorageServiceCollectionExtensions
{
/// <summary>
/// Adds the <see cref="ILocalStorageService" /> service to the service collection.
/// </summary>
public static IServiceCollection AddLocalStorageServices(
this IServiceCollection services) =>
services.AddSingleton<IJSInProcessRuntime>(serviceProvider =>
(IJSInProcessRuntime)serviceProvider.GetRequiredService<IJSRuntime>())
.AddSingleton<ILocalStorageService, LocalStorageService>();
}
using System.ComponentModel;
namespace System.Runtime.CompilerServices
{
[EditorBrowsable(EditorBrowsableState.Never)]
internal class IsExternalInit { }
}
Usefull
Download Example (.NET C# )
Share Blazorators
https://ignatandrei.github.io/RSCG_Examples/v2/docs/Blazorators